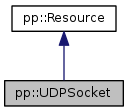
Public Member Functions
UDPSocket () | |
UDPSocket (const InstanceHandle &instance) | |
UDPSocket (PassRef, PP_Resource resource) | |
UDPSocket (const UDPSocket &other) | |
virtual | ~UDPSocket () |
UDPSocket & | operator= (const UDPSocket &other) |
int32_t | Bind (const NetAddress &addr, const CompletionCallback &callback) |
NetAddress | GetBoundAddress () |
int32_t | RecvFrom (char *buffer, int32_t num_bytes, const CompletionCallbackWithOutput< NetAddress > &callback) |
int32_t | SendTo (const char *buffer, int32_t num_bytes, const NetAddress &addr, const CompletionCallback &callback) |
void | Close () |
int32_t | SetOption (PP_UDPSocket_Option name, const Var &value, const CompletionCallback &callback) |
int32_t | JoinGroup (const NetAddress &group, const CompletionCallback callback) |
int32_t | LeaveGroup (const NetAddress &group, const CompletionCallback callback) |
Static Public Member Functions
static bool | IsAvailable () |
Detailed Description
The UDPSocket
class provides UDP socket operations.
Permissions: Apps permission socket
with subrule udp-bind
is required for Bind()
; subrule udp-send-to
is required for SendTo()
. For more details about network communication permissions, please see: /apps/app_network.html
Constructor & Destructor Documentation
pp::UDPSocket::UDPSocket | ( | const InstanceHandle & | instance | ) | [explicit] |
A constructor used to create a UDPSocket
object.
- Parameters:
[in] instance The instance with which this resource will be associated.
pp::UDPSocket::UDPSocket | ( | PassRef | , |
PP_Resource | resource | ||
) |
A constructor used when you have received a PP_Resource
as a return value that has had 1 ref added for you.
- Parameters:
[in] resource A PPB_UDPSocket
resource.
pp::UDPSocket::UDPSocket | ( | const UDPSocket & | other | ) |
virtual pp::UDPSocket::~UDPSocket | ( | ) | [virtual] |
The destructor.
Member Function Documentation
int32_t pp::UDPSocket::Bind | ( | const NetAddress & | addr, |
const CompletionCallback & | callback | ||
) |
Binds the socket to the given address.
- Parameters:
[in] addr A NetAddress
object.[in] callback A CompletionCallback
to be called upon completion.
- Returns:
- An int32_t containing an error code from
pp_errors.h
.PP_ERROR_NOACCESS
will be returned if the caller doesn't have required permissions.PP_ERROR_ADDRESS_IN_USE
will be returned if the address is already in use.
void pp::UDPSocket::Close | ( | ) |
Cancels all pending reads and writes, and closes the socket.
Any pending callbacks will still run, reporting PP_ERROR_ABORTED
if pending IO was interrupted. After a call to this method, no output paramters passed into previous RecvFrom()
calls will be accessed. It is not valid to call Bind()
again.
The socket is implicitly closed if it is destroyed, so you are not required to call this method.
Get the address that the socket is bound to.
The socket must be bound.
- Returns:
- A
NetAddress
object. The object will be null (i.e., is_null() returns true) on failure.
static bool pp::UDPSocket::IsAvailable | ( | ) | [static] |
Static function for determining whether the browser supports the PPB_UDPSocket
interface.
- Returns:
- true if the interface is available, false otherwise.
int32_t pp::UDPSocket::JoinGroup | ( | const NetAddress & | group, |
const CompletionCallback | callback | ||
) |
Joins the multicast group with address specified by group
parameter, which is expected to be a NetAddress
object.
- Parameters:
[in] group A NetAddress
corresponding to the network address of the multicast group.[in] callback A CompletionCallback
to be called upon completion.
- Returns:
- An int32_t containing an error code from
pp_errors.h
.
int32_t pp::UDPSocket::LeaveGroup | ( | const NetAddress & | group, |
const CompletionCallback | callback | ||
) |
Leaves the multicast group with address specified by group
parameter, which is expected to be a NetAddress
object.
- Parameters:
[in] group A NetAddress
corresponding to the network address of the multicast group.[in] callback A CompletionCallback
to be called upon completion.
- Returns:
- An int32_t containing an error code from
pp_errors.h
.
int32_t pp::UDPSocket::RecvFrom | ( | char * | buffer, |
int32_t | num_bytes, | ||
const CompletionCallbackWithOutput< NetAddress > & | callback | ||
) |
Receives data from the socket and stores the source address.
The socket must be bound.
Caveat: You should be careful about the lifetime of buffer
. Typically you will use a CompletionCallbackFactory
to scope callbacks to the lifetime of your class. When your class goes out of scope, the callback factory will not actually cancel the operation, but will rather just skip issuing the callback on your class. This means that if the underlying PPB_UDPSocket
resource outlives your class, the browser will still try to write into your buffer when the operation completes. The buffer must be kept valid until then to avoid memory corruption. If you want to release the buffer while the RecvFrom()
call is still pending, you should call Close()
to ensure that the buffer won't be accessed in the future.
- Parameters:
[out] buffer The buffer to store the received data on success. It must be at least as large as num_bytes
.[in] num_bytes The number of bytes to receive. [in] callback A CompletionCallbackWithOutput
to be called upon completion.
- Returns:
- A non-negative number on success to indicate how many bytes have been received; otherwise, an error code from
pp_errors.h
.
int32_t pp::UDPSocket::SendTo | ( | const char * | buffer, |
int32_t | num_bytes, | ||
const NetAddress & | addr, | ||
const CompletionCallback & | callback | ||
) |
Sends data to a specific destination.
The socket must be bound.
- Parameters:
[in] buffer The buffer containing the data to send. [in] num_bytes The number of bytes to send. [in] addr A NetAddress
object holding the destination address.[in] callback A CompletionCallback
to be called upon completion.
- Returns:
- A non-negative number on success to indicate how many bytes have been sent; otherwise, an error code from
pp_errors.h
.PP_ERROR_NOACCESS
will be returned if the caller doesn't have required permissions.PP_ERROR_INPROGRESS
will be returned if the socket is busy sending. The caller should wait until a pending send completes before retrying.
int32_t pp::UDPSocket::SetOption | ( | PP_UDPSocket_Option | name, |
const Var & | value, | ||
const CompletionCallback & | callback | ||
) |
Sets a socket option on the UDP socket.
Please see the PP_UDPSocket_Option
description for option names, value types and allowed values.
- Parameters:
[in] name The option to set. [in] value The option value to set. [in] callback A CompletionCallback
to be called upon completion.
- Returns:
- An int32_t containing an error code from
pp_errors.h
.
The documentation for this class was generated from the following file: