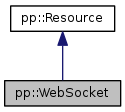
Public Member Functions
WebSocket (const InstanceHandle &instance) | |
virtual | ~WebSocket () |
int32_t | Connect (const Var &url, const Var protocols[], uint32_t protocol_count, const CompletionCallback &callback) |
int32_t | Close (uint16_t code, const Var &reason, const CompletionCallback &callback) |
int32_t | ReceiveMessage (Var *message, const CompletionCallback &callback) |
int32_t | SendMessage (const Var &message) |
uint64_t | GetBufferedAmount () |
uint16_t | GetCloseCode () |
Var | GetCloseReason () |
bool | GetCloseWasClean () |
Var | GetExtensions () |
Var | GetProtocol () |
PP_WebSocketReadyState | GetReadyState () |
Var | GetURL () |
Detailed Description
The WebSocket
class providing bi-directional, full-duplex, communications over a single TCP socket.
Constructor & Destructor Documentation
pp::WebSocket::WebSocket | ( | const InstanceHandle & | instance | ) | [explicit] |
Constructs a WebSocket object.
- Parameters:
[in] instance The instance with which this resource will be associated.
virtual pp::WebSocket::~WebSocket | ( | ) | [virtual] |
Destructs a WebSocket object.
Member Function Documentation
int32_t pp::WebSocket::Close | ( | uint16_t | code, |
const Var & | reason, | ||
const CompletionCallback & | callback | ||
) |
Close() closes the specified WebSocket connection by specifying code
and reason
.
- Parameters:
[in] code The WebSocket close code. This is ignored if it is 0. PP_WEBSOCKETSTATUSCODE_NORMAL_CLOSURE
must be used for the usual case. To indicate some specific error cases, codes in the rangePP_WEBSOCKETSTATUSCODE_USER_REGISTERED_MIN
toPP_WEBSOCKETSTATUSCODE_USER_REGISTERED_MAX
, and in the rangePP_WEBSOCKETSTATUSCODE_USER_PRIVATE_MIN
toPP_WEBSOCKETSTATUSCODE_USER_PRIVATE_MAX
are available.[in] reason A Var
of string type representing the close reason. This is ignored if it is an undefined type.[in] callback A CompletionCallback
called when the connection is closed or an error occurs in closing the connection.
- Returns:
- An int32_t containing an error code from
pp_errors.h
. ReturnsPP_ERROR_BADARGUMENT
ifreason
contains an invalid character as a UTF-8 string, or is longer than 123 bytes.PP_ERROR_BADARGUMENT
corresponds to a JavaScript SyntaxError in the WebSocket API specification. ReturnsPP_ERROR_NOACCESS
if the code is not an integer equal to 1000 or in the range 3000 to 4999.PP_ERROR_NOACCESS
corresponds to an InvalidAccessError in the WebSocket API specification. ReturnsPP_ERROR_INPROGRESS
if a previous call to Close() is not finished.
int32_t pp::WebSocket::Connect | ( | const Var & | url, |
const Var | protocols[], | ||
uint32_t | protocol_count, | ||
const CompletionCallback & | callback | ||
) |
Connect() connects to the specified WebSocket server.
You can call this function once for an object.
- Parameters:
[in] url A Var
of string type representing a WebSocket server URL.[in] protocols A pointer to an array of Var
of string type specifying sub-protocols. EachVar
represents one sub-protocol. This argument can be null only ifprotocol_count
is 0.[in] protocol_count The number of sub-protocols in protocols
.[in] callback A CompletionCallback
called when a connection is established or an error occurs in establishing connection.
- Returns:
- An int32_t containing an error code from
pp_errors.h
. ReturnsPP_ERROR_BADARGUMENT
if specifiedurl
, orprotocols
contains invalid string as defined in the WebSocket API specification.PP_ERROR_BADARGUMENT
corresponds to a SyntaxError in the WebSocket API specification. ReturnsPP_ERROR_NOACCESS
if the protocol specified in theurl
is not a secure protocol, but the origin of the caller has a secure scheme. Also returnsPP_ERROR_NOACCESS
if the port specified in theurl
is a port that the user agent is configured to block access to because it is a well-known port like SMTP.PP_ERROR_NOACCESS
corresponds to a SecurityError of the specification. ReturnsPP_ERROR_INPROGRESS
if this is not the first call to Connect().
uint64_t pp::WebSocket::GetBufferedAmount | ( | ) |
GetBufferedAmount() returns the number of bytes of text and binary messages that have been queued for the WebSocket connection to send, but have not been transmitted to the network yet.
- Returns:
- Returns the number of bytes.
uint16_t pp::WebSocket::GetCloseCode | ( | ) |
GetCloseCode() returns the connection close code for the WebSocket connection.
- Returns:
- Returns 0 if called before the close code is set.
GetCloseReason() returns the connection close reason for the WebSocket connection.
- Returns:
- Returns a
Var
of string type. If called before the close reason is set, the return value contains an empty string. Returns aPP_VARTYPE_UNDEFINED
if called on an invalid resource.
GetCloseWasClean() returns if the connection was closed cleanly for the specified WebSocket connection.
- Returns:
- Returns
false
if called before the connection is closed, called on an invalid resource, or closed for abnormal reasons. Otherwise, returnstrue
if the connection was closed cleanly.
GetExtensions() returns the extensions selected by the server for the specified WebSocket connection.
GetProtocol() returns the sub-protocol chosen by the server for the specified WebSocket connection.
PP_WebSocketReadyState pp::WebSocket::GetReadyState | ( | ) |
GetReadyState() returns the ready state of the specified WebSocket connection.
- Returns:
- Returns
PP_WEBSOCKETREADYSTATE_INVALID
if called before Connect() is called, or if this function is called on an invalid resource.
int32_t pp::WebSocket::ReceiveMessage | ( | Var * | message, |
const CompletionCallback & | callback | ||
) |
ReceiveMessage() receives a message from the WebSocket server.
This interface only returns a single message. That is, this interface must be called at least N times to receive N messages, no matter the size of each message.
- Parameters:
[out] message The received message is copied to provided message
. Themessage
must remain valid until ReceiveMessage() completes. Its receivedVar
will be of string or ArrayBuffer type.[in] callback A CompletionCallback
called when ReceiveMessage() completes. This callback is ignored if ReceiveMessage() completes synchronously and returnsPP_OK
.
- Returns:
- An int32_t containing an error code from
pp_errors.h
. If an error is detected or connection is closed, ReceiveMessage() returnsPP_ERROR_FAILED
after all buffered messages are received. Until buffered message become empty, ReceiveMessage() continues to returnPP_OK
as if connection is still established without errors.
int32_t pp::WebSocket::SendMessage | ( | const Var & | message | ) |
SendMessage() sends a message to the WebSocket server.
- Parameters:
[in] message A message to send. The message is copied to an internal buffer, so the caller can free message
safely after returning from the function. ThisVar
must be of string or ArrayBuffer types.
- Returns:
- An int32_t containing an error code from
pp_errors.h
. ReturnsPP_ERROR_FAILED
if the ReadyState isPP_WEBSOCKETREADYSTATE_CONNECTING
.PP_ERROR_FAILED
corresponds to a JavaScript InvalidStateError in the WebSocket API specification. ReturnsPP_ERROR_BADARGUMENT
if the providedmessage
contains an invalid character as a UTF-8 string.PP_ERROR_BADARGUMENT
corresponds to a JavaScript SyntaxError in the WebSocket API specification. Otherwise, returnsPP_OK
, but it doesn't necessarily mean that the server received the message.
The documentation for this class was generated from the following file: