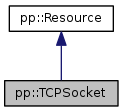
Public Member Functions
TCPSocket () | |
TCPSocket (const InstanceHandle &instance) | |
TCPSocket (PassRef, PP_Resource resource) | |
TCPSocket (const TCPSocket &other) | |
virtual | ~TCPSocket () |
TCPSocket & | operator= (const TCPSocket &other) |
int32_t | Bind (const NetAddress &addr, const CompletionCallback &callback) |
int32_t | Connect (const NetAddress &addr, const CompletionCallback &callback) |
NetAddress | GetLocalAddress () const |
NetAddress | GetRemoteAddress () const |
int32_t | Read (char *buffer, int32_t bytes_to_read, const CompletionCallback &callback) |
int32_t | Write (const char *buffer, int32_t bytes_to_write, const CompletionCallback &callback) |
int32_t | Listen (int32_t backlog, const CompletionCallback &callback) |
int32_t | Accept (const CompletionCallbackWithOutput< TCPSocket > &callback) |
void | Close () |
int32_t | SetOption (PP_TCPSocket_Option name, const Var &value, const CompletionCallback &callback) |
Static Public Member Functions
static bool | IsAvailable () |
Detailed Description
The TCPSocket
class provides TCP socket operations.
Permissions: Apps permission socket
with subrule tcp-connect
is required for Connect()
; subrule tcp-listen
is required for Listen()
. For more details about network communication permissions, please see: /apps/app_network.html
Constructor & Destructor Documentation
pp::TCPSocket::TCPSocket | ( | const InstanceHandle & | instance | ) | [explicit] |
A constructor used to create a TCPSocket
object.
- Parameters:
[in] instance The instance with which this resource will be associated.
pp::TCPSocket::TCPSocket | ( | PassRef | , |
PP_Resource | resource | ||
) |
A constructor used when you have received a PP_Resource
as a return value that has had 1 ref added for you.
- Parameters:
[in] resource A PPB_TCPSocket
resource.
pp::TCPSocket::TCPSocket | ( | const TCPSocket & | other | ) |
virtual pp::TCPSocket::~TCPSocket | ( | ) | [virtual] |
The destructor.
Member Function Documentation
int32_t pp::TCPSocket::Accept | ( | const CompletionCallbackWithOutput< TCPSocket > & | callback | ) |
Accepts a connection.
The socket must be listening.
- Parameters:
[in] callback A CompletionCallbackWithOutput
to be called upon completion.
- Returns:
- An int32_t containing an error code from
pp_errors.h
, including (but not limited to):PP_ERROR_CONNECTION_ABORTED
: A connection has been aborted.
int32_t pp::TCPSocket::Bind | ( | const NetAddress & | addr, |
const CompletionCallback & | callback | ||
) |
Binds the socket to the given address.
The socket must not be bound.
- Parameters:
[in] addr A NetAddress
object.[in] callback A CompletionCallback
to be called upon completion.
- Returns:
- An int32_t containing an error code from
pp_errors.h
, including (but not limited to):PP_ERROR_ADDRESS_IN_USE
: the address is already in use.PP_ERROR_ADDRESS_INVALID
: the address is invalid.
void pp::TCPSocket::Close | ( | ) |
Cancels all pending operations and closes the socket.
Any pending callbacks will still run, reporting PP_ERROR_ABORTED
if pending IO was interrupted. After a call to this method, no output buffer pointers passed into previous Read()
or Accept()
calls will be accessed. It is not valid to call Connect()
or Listen()
again.
The socket is implicitly closed if it is destroyed, so you are not required to call this method.
int32_t pp::TCPSocket::Connect | ( | const NetAddress & | addr, |
const CompletionCallback & | callback | ||
) |
Connects the socket to the given address.
The socket must not be listening. Binding the socket beforehand is optional.
- Parameters:
[in] addr A NetAddress
object.[in] callback A CompletionCallback
to be called upon completion.
- Returns:
- An int32_t containing an error code from
pp_errors.h
, including (but not limited to):PP_ERROR_NOACCESS
: the caller doesn't have required permissions.PP_ERROR_ADDRESS_UNREACHABLE
:addr
is unreachable.PP_ERROR_CONNECTION_REFUSED
: the connection attempt was refused.PP_ERROR_CONNECTION_FAILED
: the connection attempt failed.PP_ERROR_CONNECTION_TIMEDOUT
: the connection attempt timed out.
Since version 1.1, if the socket is listening/connected or has a pending listen/connect request, Connect()
will fail without starting a connection attempt. Otherwise, any failure during the connection attempt will cause the socket to be closed.
Gets the local address of the socket, if it is bound.
- Returns:
- A
NetAddress
object. The object will be null (i.e., is_null() returns true) on failure.
Gets the remote address of the socket, if it is connected.
- Returns:
- A
NetAddress
object. The object will be null (i.e., is_null() returns true) on failure.
static bool pp::TCPSocket::IsAvailable | ( | ) | [static] |
Static function for determining whether the browser supports the PPB_TCPSocket
interface.
- Returns:
- true if the interface is available, false otherwise.
int32_t pp::TCPSocket::Listen | ( | int32_t | backlog, |
const CompletionCallback & | callback | ||
) |
Starts listening.
The socket must be bound and not connected.
- Parameters:
[in] backlog A hint to determine the maximum length to which the queue of pending connections may grow. [in] callback A CompletionCallback
to be called upon completion.
- Returns:
- An int32_t containing an error code from
pp_errors.h
, including (but not limited to):PP_ERROR_NOACCESS
: the caller doesn't have required permissions.PP_ERROR_ADDRESS_IN_USE
: Another socket is already listening on the same port.
int32_t pp::TCPSocket::Read | ( | char * | buffer, |
int32_t | bytes_to_read, | ||
const CompletionCallback & | callback | ||
) |
Reads data from the socket.
The socket must be connected. It may perform a partial read.
Caveat: You should be careful about the lifetime of buffer
. Typically you will use a CompletionCallbackFactory
to scope callbacks to the lifetime of your class. When your class goes out of scope, the callback factory will not actually cancel the operation, but will rather just skip issuing the callback on your class. This means that if the underlying PPB_TCPSocket
resource outlives your class, the browser will still try to write into your buffer when the operation completes. The buffer must be kept valid until then to avoid memory corruption. If you want to release the buffer while the Read()
call is still pending, you should call Close()
to ensure that the buffer won't be accessed in the future.
- Parameters:
[out] buffer The buffer to store the received data on success. It must be at least as large as bytes_to_read
.[in] bytes_to_read The number of bytes to read. [in] callback A CompletionCallback
to be called upon completion.
- Returns:
- A non-negative number on success to indicate how many bytes have been read, 0 means that end-of-file was reached; otherwise, an error code from
pp_errors.h
.
int32_t pp::TCPSocket::SetOption | ( | PP_TCPSocket_Option | name, |
const Var & | value, | ||
const CompletionCallback & | callback | ||
) |
Sets a socket option on the TCP socket.
Please see the PP_TCPSocket_Option
description for option names, value types and allowed values.
- Parameters:
[in] name The option to set. [in] value The option value to set. [in] callback A CompletionCallback
to be called upon completion.
- Returns:
- An int32_t containing an error code from
pp_errors.h
.
int32_t pp::TCPSocket::Write | ( | const char * | buffer, |
int32_t | bytes_to_write, | ||
const CompletionCallback & | callback | ||
) |
Writes data to the socket.
The socket must be connected. It may perform a partial write.
- Parameters:
[in] buffer The buffer containing the data to write. [in] bytes_to_write The number of bytes to write. [in] callback A CompletionCallback
to be called upon completion.
- Returns:
- A non-negative number on success to indicate how many bytes have been written; otherwise, an error code from
pp_errors.h
.
The documentation for this class was generated from the following file: